the autocoder V241001 did a more advanced game-script
i've achieved the next milestone with the autocoder-script when creating a tic-tac-toe game.
this are the LLMs:
"OLLAMA_API_MODEL = 'deepseek-coder:33b-instruct-fp16'
OLLAMA_HELPER_API_MODEL = 'phi3:14b-medium-128k-instruct-q8_0'
OLLAMA_API_URL = 'http://localhost:11434/api'
coder_LLM = 'dolphincoder:15b-starcoder2-fp16'
coder_LLM_2 = 'yi-coder:9b-chat-fp16'"
and this the objective:
"Write a Python program that allows two players to play a game of Tic-Tac-Toe on the console. The program should start by printing out an empty 3x3 board. Players should then take turns entering their moves by specifying the row and column of the square they want to
mark. The program should validate the moves to ensure that the square is empty and that the input is within the range of the board. After each move, the program should check for a win or a tie and print out the updated board. The game should continue until one player wins or the board is full, at which point the program should declare the winner or a tie and ask if the players want to play again. The program should handle errors gracefully and allow the players to quit at any time."
this is the produced script:
"
{
"def check_win(board):"
# Check rows for win
for row in board:
if len(set(row)) == 1 and row[0] != " ":
return True
# Check columns for win
for i in range(len(board[0])):
if len(set([row[i] for row in board])) == 1 and board[0][i] != " ":
return True
# Check diagonals for win
if len(set([board[i][i] for i in range(len(board))])) == 1 and board[0][0] != " ":
return True
if len(set([board[i][len(board)-i-1] for i in range(len(board))])) == 1 and board[0][len(board)-1] != " ":
return True
return False
}
# Check if the indices are within the board dimensions
if not (0 <= row < len(board) and 0 <= col < len(board[0])):
return False
# Check if the square is empty
if board[row][col] != " " :
return False
return True"
"get_move": {
"while": "true",
"row": "int(input('Enter the row number (0, 1, or 2): '))",
"if": { "not_in": [0, 1, 2] },
"print": "Invalid input! Please enter a valid row number."
}
}
def print_board(board):
for row in board:
print(" ".join(row))
for row in board:
print(' '.join(row))
console.log(row.join(" "));
}
}
}
{
"getMove": {
"currentPlayer": ""
},
"inputValues": {
"row": 0,
"column": 0
}
}
"def is_valid_move(board, move):
row = move[0]
col = move[1]
# Check if the indices are within the board dimensions
if (row < 0 or row >= len(board)) or (col < 0 or col >= len(board[0])):
return False
# Check if the square is empty
if board[row][col] != ' ':
return False
return True"
{
"play_game": {
"player": "X",
"moves": [],
"board": {},
"is_valid_move": function() {...},
"print_board": function() {...},
# Define the function to play the game
def play_game():
board = [[" " for _ in range(3)] for _ in range(3)] # Initialize the board
player = "X"
while True:
print_board(board)
row, col = get_move()
if is_valid_move(board, row, col): # Check if move is valid
board[row][col] = player # If valid, update the board
if check_win(board, player): # Check for win condition
print_board(board)
print("Player", player, "wins!
")
break
elif all([cell != " " for row in board for cell in row]):
# If the board is full and no one won, it's a tie
print_board(board)
print("It is a tie!
")
break
player = "O" if player == "X" else "X"
else:
print("Invalid move. Please try again.")
# Define the function to check for win condition
def check_win(board, player):
# Check rows
for row in board:
if all([cell == player for cell in row]):
return True
# Check columns
for col in range(3):
if all([row[col] == player for row in board]):
return True
# Check diagonals
if all([board[i][i] == player for i in range(3)]) or all([board[i][2-i] == player for i in range(3)]):
return True
return False
# Define the function to get a valid move from the user
def get_move():
while True:
try: # Try to get a valid move
row = int(input("Enter row number (0-2): "))
col = int(input("Enter column number (0-2): "))
return row, col
except ValueError:
print("Invalid input. Please enter a number.")
# Define the function to check if a move is valid
def is_valid_move(board, row, col):
# Check if the cell is empty and inside the board
return 0 <= row < 3 and 0 <= col < 3 and board[row][col] == " "
}
}
"
because the refactoring (in the autocoder script) isn't finished jet- it needs to be adapted:
1. the game-code is completed (expect one code-line nr.4 in this case)
2. some parts of the script (double records- working versions) are deleted
"
def print_board(board):
for row in board:
print(" ".join(row))
print("-" * 9)
def play_game():
board = [[" " for _ in range(3)] for _ in range(3)] # Initialize the board
player = "X"
while True:
print_board(board)
row, col = get_move()
if is_valid_move(board, row, col): # Check if move is valid
board[row][col] = player # If valid, update the board
if check_win(board, player): # Check for win condition
print_board(board)
print("Player", player, "wins!")
break
elif all([cell != " " for row in board for cell in row]):
# If the board is full and no one won, it's a tie
print_board(board)
print("It is a tie!")
break
player = "O" if player == "X" else "X"
else:
print("Invalid move. Please try again.")
# Define the function to check for win condition
def check_win(board, player):
# Check rows
for row in board:
if all([cell == player for cell in row]):
return True
# Check columns
for col in range(3):
if all([row[col] == player for row in board]):
return True
# Check diagonals
if all([board[i][i] == player for i in range(3)]) or all([board[i][2-i] == player for i in range(3)]):
return True
return False
# Define the function to get a valid move from the user
def get_move():
while True:
try: # Try to get a valid move
row = int(input("Enter row number (0-2): "))
col = int(input("Enter column number (0-2): "))
return row, col
except ValueError:
print("Invalid input. Please enter a number.")
# Define the function to check if a move is valid
def is_valid_move(board, row, col):
# Check if the cell is empty and inside the board
return 0 <= row < 3 and 0 <= col < 3 and board[row][col] == " "
# Start the game
if __name__ == "__main__":
play_game()"
the game is created with the help of the ChatGPT, which gave a score (also correction of the script) to the corrupt pre-versions of the script and this response was given as input to the LLMs memory.
this is a mayor step for me in creation of such a coder-LLM app, get an overview of the AI landscape and learning by doing python coding.
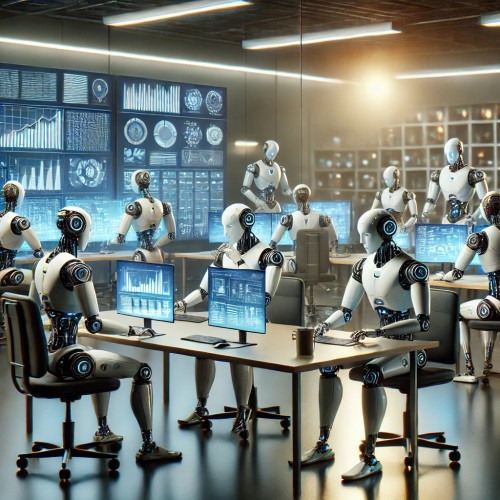
- ·
- · Dusko
- · Technology
- · dichipcoin
- · 6364 views